ARナビゲーション:
Mapbox Vision AR forは、Mapbox Vision SDKの一番上にある高レベルのフレームワークである。 Vision ARはナビゲーションルートを管理し、コアライブラリに変換し、デバイスの組み込みカメラからのライブビデオストリームの一番上にあるARナビゲーションルートをレンダリングする。
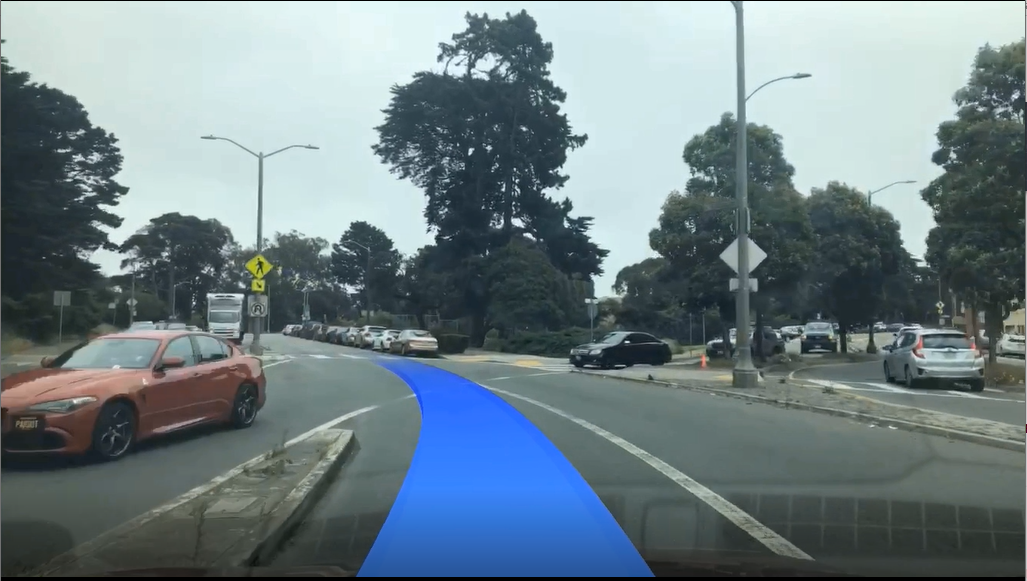
路線
iOS
ルートを生成して、道路のルートラインを投影し始める。 MapboxDirections.swiftを使用して、新しいRouteオブジェクトを作成する。これを使用して、地図上にルートを表示したり、ナビゲーションシーケンスを開始したりする。ほとんどの場合には、いくつかのオプションを設定するのにNavigationRouteOptionsクラス(MapboxDirections.swiftのRouteOptionsクラスのサブクラス) も使用される。
次に、VisionARManagerを作成して、生成したルートにルートを設定する。
1. visionARManager = VisionARManager.create(visionManager: visionManager, delegate: self)
2.
3. let origin = CLLocationCoordinate2D()
4. let destination = CLLocationCoordinate2D()
5. let options = NavigationRouteOptions(coordinates: [origin, destination], profileIdentifier: .automobile)
6.
7. // query a navigation route between location coordinates and pass it to VisionARManager
8. Directions.shared.calculate(options) { [weak self] (waypoints, routes, error) in
9. guard let route = routes?.first else { return }
10. self?.visionARManager.set(route: Route(route: route))
11. }
iOS実装の例
1. import UIKit
2. import MapboxVision
3. import MapboxVisionAR
4. import MapboxDirections
5. import MapboxCoreNavigation
6.
7. /**
8. * "AR Navigation" example demonstrates how to display navigation route projected on the surface of the road.
9. */
10.
11. class ARNavigationViewController: UIViewController {
12. private var videoSource: CameraVideoSource!
13. private var visionManager: VisionManager!
14. private var visionARManager: VisionARManager!
15.
16. private let visionARViewController = VisionARViewController()
17.
18. override func viewDidLoad() {
19. super.viewDidLoad()
20.
21. addARView()
22.
23. // create a video source obtaining buffers from camera module
24. videoSource = CameraVideoSource()
25. videoSource.add(observer: self)
26.
27. // create VisionManager with video source
28. visionManager = VisionManager.create(videoSource: videoSource)
29. // create VisionARManager and register as its delegate to receive AR related events
30. visionARManager = VisionARManager.create(visionManager: visionManager, delegate: self)
31.
32. let origin = CLLocationCoordinate2D()
33. let destination = CLLocationCoordinate2D()
34. let options = NavigationRouteOptions(coordinates: [origin, destination], profileIdentifier: .automobile)
35.
36. // query a navigation route between location coordinates and pass it to VisionARManager
37. Directions.shared.calculate(options) { [weak self] (waypoints, routes, error) in
38. guard let route = routes?.first else { return }
39. self?.visionARManager.set(route: Route(route: route))
40. }
41. }
42.
43. override func viewWillAppear(_ animated: Bool) {
44. super.viewWillAppear(animated)
45.
46. visionManager.start()
47. videoSource.start()
48. }
49.
50. override func viewDidDisappear(_ animated: Bool) {
51. super.viewDidDisappear(animated)
52.
53. videoSource.stop()
54. visionManager.stop()
55. // free up resources by destroying modules when they're not longer used
56. visionARManager.destroy()
57. }
58.
59. private func addARView() {
60. addChild(visionARViewController)
61. view.addSubview(visionARViewController.view)
62. visionARViewController.didMove(toParent: self)
63. }
64. }
65.
66. extension ARNavigationViewController: VisionARManagerDelegate {
67. func visionARManager(_ visionARManager: VisionARManager, didUpdateARCamera camera: ARCamera) {
68. DispatchQueue.main.async { [weak self] in
69. // pass the camera parameters for projection calculation
70. self?.visionARViewController.present(camera: camera)
71. }
72. }
73.
74. func visionARManager(_ visionARManager: VisionARManager, didUpdateARLane lane: ARLane?) {
75. DispatchQueue.main.async { [weak self] in
76. // display AR lane representing navigation route
77. self?.visionARViewController.present(lane: lane)
78. }
79. }
80. }
81.
82. extension ARNavigationViewController: VideoSourceObserver {
83. func videoSource(_ videoSource: VideoSource, didOutput videoSample: VideoSample) {
84. DispatchQueue.main.async { [weak self] in
85. // display received sample buffer by passing it to ar view controller
86. self?.visionARViewController.present(sampleBuffer: videoSample.buffer)
87. }
88. }
89. }