ARナビゲーション:
Mapbox Vision AR forは、Mapbox Vision SDKの一番上にある高レベルのフレームワークである。 Vision ARはナビゲーションルートを管理し、コアライブラリに変換し、デバイスの組み込みカメラからのライブビデオストリームの一番上にあるARナビゲーションルートをレンダリングする。
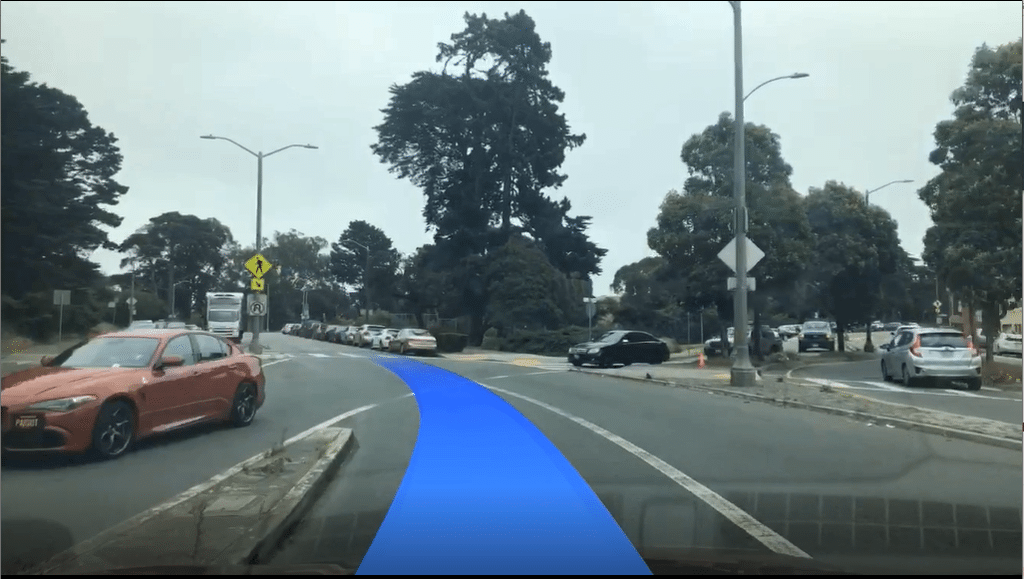
路線
Android:
ルートを生成して、道路のルートラインを投影し始める。 Mapbox Navigation SDKを使用して、Mapbox Directions APIへの新しいリクエストを作成するか、又はカスタムナビゲーションエンジンを使用する。 Android向けMapbox Navigation SDKを使用したい場合は、Navigation SDK ドキュメントの指示に従って、依存関係で追加する必要がある。
以下の例では、AndroidのNavigationRoute.BuilderクラスのAndroiMapbox Navigation SDKを使用して、Mapbox Directions APIへの新しいリクエストを作成する。リスポンスは地図上にルート表示、又はナビゲーションシーケンス開始に使用できるNavigationRouteオブジェクトになる。ナビゲーションSDKでルート生成の詳細については、ルート生成の案内書を参考してください。
VisionArManagerのsetRouteメソッドでVision ARビューで生成されたルートを使用できる。
1. VisionArManager.setRoute(new Route(
2. getRoutePoints(route),
3. (float) routeProgress.durationRemaining(),
4. "Source street name",
5. "Target street name"
6. ));
デフォルトでは、ルートを表示するのに青い実線が使用されているが、カスタマイズが可能である。 VisionArView.setLaneVisualParamsを使用して、色、幅、光の座標、光の色、環境光の色などを変更する。
Android実装の例
下記の例には、2つの追加の依存関係が必要である。 これらの依存関係をgradleファイルに追加する。
1. implementation "com.mapbox.mapboxsdk:mapbox-android-navigation:0.22.0" 2. implementation "com.mapbox.mapboxsdk:mapbox-android-core:0.2.1" 3. package com.mapbox.vision.examples; 4. 5. import android.annotation.SuppressLint; 6. import android.location.Location; 7. import android.os.Bundle; 8. import android.support.v7.app.AppCompatActivity; 9. import android.widget.Toast; 10. import com.mapbox.android.core.location.LocationEngine; 11. import com.mapbox.android.core.location.LocationEngineListener; 12. import com.mapbox.android.core.location.LocationEnginePriority; 13. import com.mapbox.android.core.location.LocationEngineProvider; 14. import com.mapbox.api.directions.v5.models.*; 15. import com.mapbox.geojson.Point; 16. import com.mapbox.services.android.navigation.v5.navigation.MapboxNavigation; 17. import com.mapbox.services.android.navigation.v5.navigation.MapboxNavigationOptions; 18. import com.mapbox.services.android.navigation.v5.navigation.NavigationRoute; 19. import com.mapbox.services.android.navigation.v5.offroute.OffRouteListener; 20. import com.mapbox.services.android.navigation.v5.route.RouteFetcher; 21. import com.mapbox.services.android.navigation.v5.route.RouteListener; 22. import com.mapbox.services.android.navigation.v5.routeprogress.ProgressChangeListener; 23. import com.mapbox.services.android.navigation.v5.routeprogress.RouteProgress; 24. import com.mapbox.vision.VisionManager; 25. import com.mapbox.vision.ar.VisionArManager; 26. import com.mapbox.vision.ar.core.models.Route; 27. import com.mapbox.vision.ar.core.models.RoutePoint; 28. import com.mapbox.vision.ar.view.gl.VisionArView; 29. import com.mapbox.vision.mobile.core.interfaces.VisionEventsListener; 30. import com.mapbox.vision.mobile.core.models.AuthorizationStatus; 31. import com.mapbox.vision.mobile.core.models.Camera; 32. import com.mapbox.vision.mobile.core.models.Country; 33. import com.mapbox.vision.mobile.core.models.FrameSegmentation; 34. import com.mapbox.vision.mobile.core.models.classification.FrameSignClassifications; 35. import com.mapbox.vision.mobile.core.models.detection.FrameDetections; 36. import com.mapbox.vision.mobile.core.models.position.GeoCoordinate; 37. import com.mapbox.vision.mobile.core.models.position.VehicleState; 38. import com.mapbox.vision.mobile.core.models.road.RoadDescription; 39. import com.mapbox.vision.mobile.core.models.world.WorldDescription; 40. import com.mapbox.vision.performance.ModelPerformance.On; 41. import com.mapbox.vision.performance.ModelPerformanceConfig.Merged; 42. import com.mapbox.vision.performance.ModelPerformanceMode; 43. import com.mapbox.vision.performance.ModelPerformanceRate; 44. import org.jetbrains.annotations.NotNull; 45. import retrofit2.Call; 46. import retrofit2.Callback; 47. import retrofit2.Response; 48. 49. import java.util.ArrayList; 50. import java.util.List; 51. 52. /** 53. * Example shows how Vision and VisionAR SDKs are used to draw AR lane over the video stream from camera. 54. * Also, Mapbox navigation services are used to build route and navigation session. 55. */ 56. public class ArActivity extends AppCompatActivity implements LocationEngineListener, RouteListener, ProgressChangeListener, OffRouteListener { 57. 58. // Handles navigation. 59. private MapboxNavigation mapboxNavigation; 60. // Fetches route from points. 61. private RouteFetcher routeFetcher; 62. private RouteProgress lastRouteProgress; 63. private DirectionsRoute directionsRoute; 64. private LocationEngine arLocationEngine; 65. 66. // This dummy points will be used to build route. For real world test this needs to be changed to real values for 67. // source and target locations. 68. private final Point ROUTE_ORIGIN = Point.fromLngLat(35.681461, 139.766357); 69. private final Point ROUTE_DESTINATION = Point.fromLngLat(35.686468, 139.716307); 70. 71. @Override 72. protected void onCreate(Bundle savedInstanceState) { 73. super.onCreate(savedInstanceState); 74. this.setContentView(R.layout.activity_ar_navigation); 75. 76. // Initialize navigation with your Mapbox access token. 77. mapboxNavigation = new MapboxNavigation( 78. this, 79. getString(R.string.mapbox_access_token), 80. MapboxNavigationOptions.builder().enableOffRouteDetection(true).build() 81. ); 82. 83. // Initialize route fetcher with your Mapbox access token. 84. routeFetcher = new RouteFetcher(this, getString(R.string.mapbox_access_token)); 85. routeFetcher.addRouteListener(this); 86. 87. LocationEngineProvider provider = new LocationEngineProvider(this); 88. arLocationEngine = provider.obtainBestLocationEngineAvailable(); 89. arLocationEngine.setPriority(LocationEnginePriority.HIGH_ACCURACY); 90. arLocationEngine.setInterval(0); 91. arLocationEngine.setFastestInterval(1000); 92. } 93. 94. @Override 95. protected void onResume() { 96. super.onResume(); 97. arLocationEngine.activate(); 98. arLocationEngine.addLocationEngineListener(this); 99. 100. initDirectionsRoute(); 101. 102. // Route need to be reestablished if off route happens. 103. mapboxNavigation.addOffRouteListener(this); 104. mapboxNavigation.addProgressChangeListener(this); 105. 106. // Create and start VisionManager. 107. VisionManager.create(); 108. VisionManager.setModelPerformanceConfig(new Merged(new On(ModelPerformanceMode.FIXED, ModelPerformanceRate.LOW))); 109. VisionManager.start( 110. new VisionEventsListener() { 111. 112. @Override 113. public void onAuthorizationStatusUpdated(@NotNull AuthorizationStatus authorizationStatus) {} 114. 115. @Override 116. public void onFrameSegmentationUpdated(@NotNull FrameSegmentation frameSegmentation) {} 117. 118. @Override 119. public void onFrameDetectionsUpdated(@NotNull FrameDetections frameDetections) {} 120. 121. @Override 122. public void onFrameSignClassificationsUpdated(@NotNull FrameSignClassifications frameSignClassifications) {} 123. 124. @Override 125. public void onRoadDescriptionUpdated(@NotNull RoadDescription roadDescription) {} 126. 127. @Override 128. public void onWorldDescriptionUpdated(@NotNull WorldDescription worldDescription) {} 129. 130. @Override 131. public void onVehicleStateUpdated(@NotNull VehicleState vehicleState) {} 132. 133. @Override 134. public void onCameraUpdated(@NotNull Camera camera) {} 135. 136. @Override 137. public void onCountryUpdated(@NotNull Country country) {} 138. 139. @Override 140. public void onUpdateCompleted() {} 141. } 142. ); 143. 144. VisionArView visionArView = findViewById(R.id.mapbox_ar_view); 145. VisionManager.setVideoSourceListener(visionArView); 146. 147. // Create VisionArManager. 148. VisionArManager.create(VisionManager.INSTANCE, visionArView); 149. } 150. 151. private void initDirectionsRoute() { 152. // Get route from predefined points. 153. NavigationRoute.builder(this) 154. .accessToken(getString(R.string.mapbox_access_token)) 155. .origin(ROUTE_ORIGIN) 156. .destination(ROUTE_DESTINATION) 157. .build() 158. .getRoute(new Callback() { 159. @Override 160. public void onResponse(Call call, Response response) { 161. if (response.body() == null || response.body().routes().isEmpty()) { 162. return; 163. } 164. 165. // Start navigation session with retrieved route. 166. DirectionsRoute route = response.body().routes().get(0); 167. mapboxNavigation.startNavigation(route); 168. 169. // Set route progress. 170. VisionArManager.setRoute(new Route( 171. getRoutePoints(route), 172. directionsRoute.duration().floatValue(), 173. "", 174. "" 175. )); 176. } 177. 178. @Override 179. public void onFailure(Call call, Throwable t) {} 180. }); 181. } 182. 183. @Override 184. protected void onPause() { 185. super.onPause(); 186. VisionArManager.destroy(); 187. VisionManager.stop(); 188. VisionManager.destroy(); 189. 190. arLocationEngine.removeLocationUpdates(); 191. arLocationEngine.removeLocationEngineListener((LocationEngineListener) this); 192. arLocationEngine.deactivate(); 193. 194. mapboxNavigation.removeProgressChangeListener(this); 195. mapboxNavigation.removeOffRouteListener(this); 196. mapboxNavigation.stopNavigation(); 197. } 198. 199. @Override 200. public void onLocationChanged(Location location) {} 201. 202. @Override 203. @SuppressLint({"MissingPermission"}) 204. public void onConnected() { 205. arLocationEngine.requestLocationUpdates(); 206. } 207. 208. @Override 209. public void onErrorReceived(Throwable throwable) { 210. if (throwable != null) { 211. throwable.printStackTrace(); 212. } 213. 214. mapboxNavigation.stopNavigation(); 215. Toast.makeText(this, "Can not calculate the route requested", Toast.LENGTH_SHORT).show(); 216. } 217. 218. @Override 219. public void onResponseReceived(@NotNull DirectionsResponse response, RouteProgress routeProgress) { 220. mapboxNavigation.stopNavigation(); 221. if (response.routes().isEmpty()) { 222. Toast.makeText(this, "Can not calculate the route requested", Toast.LENGTH_SHORT).show(); 223. } else { 224. DirectionsRoute route = response.routes().get(0); 225. 226. mapboxNavigation.startNavigation(route); 227. 228. // Set route progress. 229. VisionArManager.setRoute(new Route( 230. getRoutePoints(route), 231. (float) routeProgress.durationRemaining(), 232. "", 233. "" 234. )); 235. } 236. } 237. 238. @Override 239. public void onProgressChange(Location location, RouteProgress routeProgress) { 240. lastRouteProgress = routeProgress; 241. } 242. 243. @Override 244. public void userOffRoute(Location location) { 245. routeFetcher.findRouteFromRouteProgress(location, lastRouteProgress); 246. } 247. 248. private RoutePoint[] getRoutePoints(@NotNull DirectionsRoute route) { 249. ArrayList routePoints = new ArrayList<>(); 250. 251. List legs = route.legs(); 252. if (legs != null) { 253. for (RouteLeg leg : legs) { 254. 255. List steps = leg.steps(); 256. if (steps != null) { 257. for (LegStep step : steps) { 258. RoutePoint point = new RoutePoint((new GeoCoordinate( 259. step.maneuver().location().latitude(), 260. step.maneuver().location().longitude() 261. ))); 262. 263. routePoints.add(point); 264. 265. List intersections = step.intersections(); 266. if (intersections != null) { 267. for (StepIntersection intersection : intersections) { 268. point = new RoutePoint((new GeoCoordinate( 269. step.maneuver().location().latitude(), 270. step.maneuver().location().longitude() 271. ))); 272. 273. routePoints.add(point); 274. } 275. } 276. } 277. } 278. } 279. } 280. 281. return routePoints.toArray(new RoutePoint[0]); 282. } 283. }