安全警告
開発者は、アドオンモジュールであるMapbox Vision Safetyを使用して、道路状況と潜在的な危険を運転手に通知・警告する機能を作成できる。(Mapbox Vision Safety がVision SDKから渡されたセグメンテーション、検出、および分類情報を使用する)
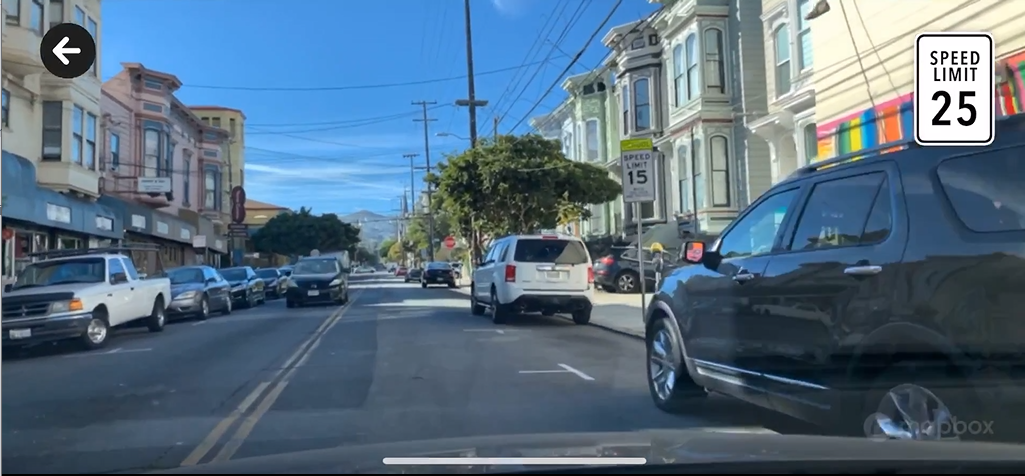
速度制限の監視
開発者は、標識分類を使用して速度制限および他の重要な標識を監視したり、最近によく観察された速度制限を追跡したりすることができる。車両の速度が最近の観察された速度制限を超えた場合、プログラム的なアラートを設定できる。
速度警告は、速度制限標識と車両の現在速度標識という2つのサインがある。
標識の検出
Vision SafetyがMapbox Vision SDKのDetectionClass を使用して、表示されているtrafficSignsを検出して、speedLimit*タイプ(トラックの最高速度や最低速度などの特別な速度制限の標識を含む)に基づいてSignTypesにフィルターする。
Vision SafetyのSpeedLimitRangeクラスには最小および最大の速度制限の数値が含まれている。
車両速度
Vision SDKでのVehicleLocationクラスの速度プロパティを使用して、デバイスの現在速度を判断する。
次に、現在の車両速度を速度制限範囲と比較して、適当な速度通知をトリガーできる。
iOS実装の例:
1. import Foundation
2. import UIKit
3. import MapboxVision
4. import MapboxVisionSafety
5.
6. /**
7. * "Over speeding" example demonstrates how to utilize events from MapboxVisionSafetyManager to alert a user about exceeding allowed speed limit.
8. */
9.
10. class OverSpeedingViewController: UIViewController {
11. private var videoSource: CameraVideoSource!
12. private var visionManager: VisionManager!
13. private var visionSafetyManager: VisionSafetyManager!
14.
15. private let visionViewController = VisionPresentationViewController()
16. private var alertView: UIView!
17.
18. private var vehicleState: VehicleState?
19. private var restrictions: RoadRestrictions?
20.
21. override func viewDidLoad() {
22. super.viewDidLoad()
23.
24. addVisionView()
25. addAlertView()
26.
27. // create a video source obtaining buffers from camera module
28. videoSource = CameraVideoSource()
29. videoSource.add(observer: self)
30.
31. // create VisionManager with video source
32. visionManager = VisionManager.create(videoSource: videoSource)
33. // create VisionSafetyManager and register as its delegate to receive safety related events
34. visionSafetyManager = VisionSafetyManager.create(visionManager: visionManager, delegate: self)
35. }
36.
37. override func viewWillAppear(_ animated: Bool) {
38. super.viewWillAppear(animated)
39.
40. visionManager.start(delegate: self)
41. videoSource.start()
42. }
43.
44. override func viewDidDisappear(_ animated: Bool) {
45. super.viewDidDisappear(animated)
46.
47. videoSource.stop()
48. visionManager.stop()
49. // free up resources by destroying modules when they're not longer used
50. visionSafetyManager.destroy()
51. }
52.
53. private func addVisionView() {
54. addChild(visionViewController)
55. view.addSubview(visionViewController.view)
56. visionViewController.didMove(toParent: self)
57. }
58.
59. private func addAlertView() {
60. alertView = UIImageView(image: UIImage(named: "alert"))
61. alertView.isHidden = true
62. alertView.translatesAutoresizingMaskIntoConstraints = false
63. view.addSubview(alertView)
64. NSLayoutConstraint.activate([
65. alertView.topAnchor.constraint(equalToSystemSpacingBelow: view.topAnchor, multiplier: 1),
66. view.trailingAnchor.constraint(equalToSystemSpacingAfter: alertView.trailingAnchor, multiplier: 1)
67. ])
68. }
69. }
70.
71. extension OverSpeedingViewController: VisionManagerDelegate, VisionSafetyManagerDelegate {
72. func visionManager(_ visionManager: VisionManager, didUpdateVehicleState vehicleState: VehicleState) {
73. DispatchQueue.main.async { [weak self] in
74. // save the latest state of the vehicle
75. self?.vehicleState = vehicleState
76. }
77. }
78.
79. func visionSafetyManager(_ visionSafetyManager: VisionSafetyManager, didUpdateRoadRestrictions roadRestrictions: RoadRestrictions) {
80. DispatchQueue.main.async { [weak self] in
81. // save currenly applied road restrictions
82. self?.restrictions = roadRestrictions
83. }
84. }
85.
86. func visionManagerDidCompleteUpdate(_ visionManager: VisionManager) {
87. DispatchQueue.main.async { [weak self] in
88. // when update is completed all the data has the most current state
89. guard let state = self?.vehicleState, let restrictions = self?.restrictions else { return }
90.
91. // decide whether speed limit is exceeded by comparing it with the current speed
92. let isOverSpeeding = state.speed > restrictions.speedLimits.speedLimitRange.max
93. self?.alertView.isHidden = !isOverSpeeding
94. }
95. }
96. }
97.
98. extension OverSpeedingViewController: VideoSourceObserver {
99. public func videoSource(_ videoSource: VideoSource, didOutput videoSample: VideoSample) {
100. DispatchQueue.main.async { [weak self] in
101. // display received sample buffer by passing it to presentation controller
102. self?.visionViewController.present(sampleBuffer: videoSample.buffer)
103. }
104. }
105. }